Looking better
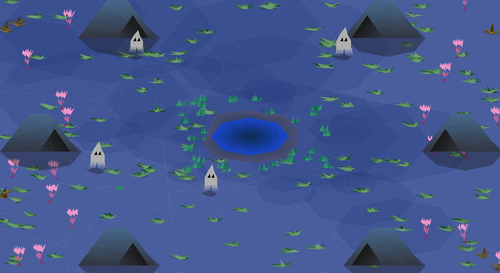
I spent more time after the stream the other day tweaking the plants and caves, setting specific colors , adding little details like ground "splotches" and shadows beneath the rock homes.
Today I spent time implementing animation, which meant that I needed to add a way to mark when vertices are "connected" to each other so that if a vertex moves, any other vertices attached to it also move. It took some tweaking, but I made a VertexConnection class:
class VertexConnection
{
public:
VertexConnection()
{
baseIndex = -1;
}
int baseIndex;
std::vector<int> connectionIndices;
};
I originally wrote it to point to specific vertices but it seems like the addresses of those vertices in the vertex array changed after set up. The vertex array is a member of the class, so it shouldn't be changing its contents (I would think?) but I did a workaround where I referenced the indices instead. During the setup of creating the graphics, I'd keep track of what vertex was connecting to another, and add it into the ProceduralObject class family's std::map<int, VertexConnection> m_vertexConnections;
like this:
tipIndex = m_vertexArrays[0].getVertexCount() - 1;
m_vertexConnections[tipIndex].baseIndex = tipIndex;
Then, when the object's update function is ran, different animations can be done by manipulating x,y coordinates. For example, the tree coral just shifts a pixel in either direction:
void TreeCoral::Update()
{
IncrementFrame();
if ( m_currentFrame == 0 )
{
for ( auto& v : m_vertexConnections )
{
float xChange = chalo::GetRandomNumber( -1, 1 );
float yChange = chalo::GetRandomNumber( -1, 1 );
m_vertexArrays[0][v.second.baseIndex].position.x += xChange;
m_vertexArrays[0][v.second.baseIndex].position.y += yChange;
for ( auto& c : v.second.connectionIndices )
{
m_vertexArrays[0][c].position = m_vertexArrays[0][v.second.baseIndex].position;
}
}
}
}
This works pretty well and I was able to add animations to each of the plant types and I made a slight bobbing animation for the cephalopods (who I really need to name...). The result is this:
So now, still building all the graphics out of triangles procedurally, I think that I have an actually decent looking aesthetic. This was my first challenge - to try to build a scene to try to come up with an idea of what the game would look like - would I even want to go with no bitmap sprites for graphics? And I think I'm pretty happy with this. I can continue expanding and adding onto the game by adding additional children of the ProceduralObject class, I don't have to do a bunch of spritework, and also playing around with vertices gives me a chance to explore and experiment, making the coding process more fun for me.
Mupoa: Bo Pu - To The Unknown
Status | In development |
Author | Moosadee |
More posts
- Gamedev Stream - Caves and JellyfishMay 21, 2021
- Teh graphics R uglyMay 20, 2021
- But what IS the gameplay...?May 20, 2021
- Designing on paperMay 01, 2021
Leave a comment
Log in with itch.io to leave a comment.